CS46A Lab
Two-Dimensional Arrays
Copyright © Cay S. Horstmann, Kathleen O’Brien 2009-2014 
This work is licensed under a Creative Commons Attribution-ShareAlike 4.0 International License.
Part A. Patterns
- Look at the (incomplete) code in Pattern.java class. This class has a 2-D array of Color objects as an instance variable.
private Color[][] colors;
The constructor instantiates the array. Note how the makePattern
method traverses the 2-D array. When the ... is replaced with the correct code, it fills the 2-D array with Color objects. It fills all the squares in the first row, then all the squares in the second row ...
Scribe: What happens if you replace the ... with Color.GREEN?
This program uses the graphics from the Java library. That's okay. You only need to consider the makePattern
method and how to traverse the 2-D array of Color objects. You should not import Dr Horstmann's graphics package.
- Provide pseudocode for creating this pattern:
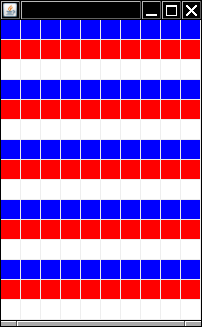
Ask yourselves: (Scribe: Record your answers)
- How would you make one row blue
- How do you make all the blue rows?
- At what index values do you make all the squares in a row blue? (think %)
- What if statement could you use to determine what color the row should be?
- Implement the code and execute it. Driver: Put the code of the
makePattern
method in your lab report.
- Each of you comes up with a pattern that you want your partner to implement. Sketch the pattern on a sheet of paper. Be creative, but keep in mind that the pattern should be regular enough that it can be done with a couple of loops.
- If either of you feels that the pattern you got from the other is too hard to code, you can refuse it. In that case, each of you implements the pattern that you designed. So be sure you can implement your own design.
- Discuss the pattern that you got from your partner and be sure you know what your partner wants. Ask for clarification if necessary.
- Implement the pattern. Scribe and Driver: Upload a screen capture along with your report. Paste the code to your lab report.
Part B. The Negative of a Picture
- There are going to be several exercises working with pictures. Make a new BlueJ project, and import Dr. Horstmann's graphics library.
- The first part of this exercise creates a new Picture object in gray tones using only the colors black and white. A picture is represented by a two-dimensional array of pixels. If the picture is black and white, each array element can be an integer representing the grayscale value. There are two useful features for our purposes in the Picture class:
- public int[][] getGrayLevels() is a method which returns a 2-D int array where each element contains the gray scale value (between 0 and 255) of the correspondng pixel in the picture.
- public Picture(int[][] grayLevels)is a constructor that takes a 2-D int array and creates a Picture object which can be displayed. (Note that the grayscale 2-D array is a representation of the Picture but not a Picture object. This constructor creates a Picture object from the array)
- Create a class PictureViewer and paste in the following code:
public class PictureViewer
{
public static void main(String[] args)
{
Picture blimp = new Picture("blimp.jpg");
int[][] grayscale = blimp.getGrayLevels();
Picture grayBlimp = new Picture(grayscale);
grayBlimp.draw();
}
}
Save the image below into the project directory. Run the program. Scribe: What happens?
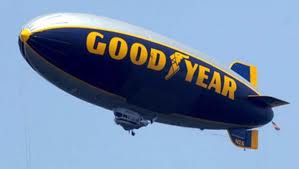
- Now we want to form a negative of this picture in grayscale. To do that, for each value (call it
c
) in the 2-D array, calculate 255 - c
(which will be the "negative"), and store it back into the array at the same location. Write the code that does this replacement for all values (pixels) in the 2-D grayscale array. (This code goes in the PictureViewer class Do not modify the Picture class). You will need nested loops, one to go through each row and one to go through each column in the row. . You will pick up each element in the array, tranform it to its negative and save it back in the array. Scribe: How many rows are in the array? Scribe: How many columns?Driver: What is your code?
- The array represents the picture but is not a Picture object. This statement from step 3 created a new Picture object from the array by using the contructor that takes a grayscale int[][].
Picture grayBlimp = new Picture(grayscale)
Compile and run the code. Scribe: What happens when you run the program?
Part C. Reflection
- Now we want to reflect a picture along the vertical axis. Make a new class ReflectionViewer and copy the main method from PictureViewer into it.
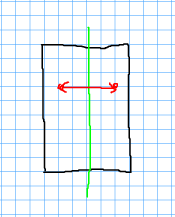
Assume that the image is 100 pixels wide and 200 pixels high. Scribe: Where does pixels[0][0] go when reflected?
- Scribe:Where does the top right corner go?
- Scribe: In general, to which row and column does pixel[i][j] go when reflected? (Do not skip this step)
- Scribe: What is wrong with this pseudocode for reflecting the image?
for i from 0 to rows - 1
for j from 0 to columns - 1
i2 = target row (as determined in step 3)
j2 = target column (as determined in step 3)
pixels[i2][j2] = pixels[i][j]
pixels[i][j] = pixels[i2][j2]
- Scribe: What is wrong with this pseudocode for reflecting the image?
for i from 0 until rows - 1
for j from 0 until columns - 1
i2 = target row (as in step 3)
j2 = target column (as in step 3)
swap pixels[i][j] with pixels[i2][j2]
Fix the pseudocode.
- Implement the code that carries out the reflection. Driver: What is the code?
- Run your program. Scribe: What happens?
Part D. Rotating a picture
- Now we want to rotate the picture by 90 degrees clockwise. Make a class called RotateViewer and copy the main method from the PictureViewer into it. Take out a piece of graph paper and make a sketch of an image and its rotation.
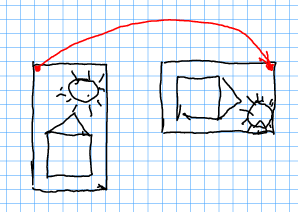
Assume the original image is 100 pixels wide and 200 pixels high. Scribe: What is the width and height of the rotated image?
- Scribe: Where does pixels[0][0] go when rotated?
- Scribe: Where does the top right corner go?
- Scribe: In general, to which row and column does pixel[i][j] go when rotated? (Do not skip this step! If you can't figure it out, ask your lab instructor.)
- When we created the the negative and reflection , we were able to edit the image in place. Scribe: Can you similarly write code so that afterwards, the
pixels
array contains the rotated image? Why or why not?
- You will need to implement the code so a new array containing the rotated image is created, without modifying the original.
- First, construct a new array of the correct size. Driver: How do you do that?
- To create the rotated Picture, you will need to iterate through every element in the original Picture and put that pixel in the correct position in the rotated Picture. You will use two nested loops to put
pixels[i][j]
into the correct places of the array of rotated Picture. Driver: What is your code?
- Run your program. Scribe: What happens?