CS46A Lab
More with Existing Classes
Copyright © Cay S. Horstmann, Kathleen O’Brien 2009-2016 
This work is licensed under a Creative Commons Attribution-ShareAlike 4.0 International License.
Instructions
- You work in pairs.
- One of you is the "scribe", the other the "driver" who runs the tools. Only the driver will write code
- The scribe answers the questions, except for the questions that say "paste the code"—the driver does the pasting.
- Next lab, you switch roles. You must alternate between Driver and Scribe roles.
- Scribe: Start writing a report with the title "Scribe's name (Scribe) / driver's name (Driver)", for example
Lab 1 Fred Foo (Scribe) / Becky Bar (Driver)
- Driver: Start writing a report with the title "Driver's name (Driver) / scribe's name (Scribe)", for example
Lab 1 Becky Bar (Driver) / Fred Foo (Scribe)
- When a step contains a question, the scribe puts the answer in the scribe report. Otherwise, just put "Step n: done"
- When a step asks "what is your code", the driver pastes the code into the driver report.
- Stuck? Discuss with your buddy, and if you are both stuck, ask for help!!!
- Read these lab rules
Note: This lab looks similar to lab 2 but it is different. In lab 2 you used the workbench. In this lab you will do the same thing in code. Rather than make a new folder and new Bluej projects for this lab, you can just use the projects in the lab2 folder and add code to those classes.
Part A
- Last lab you created objects and called their methods using the Bluej workbench and in the code pad. Now let's do the same thing in code. The advantage to using code is that you can do the same thing over and over with little extra work. Or you can give the program to someone else to run.
- The driver for this week needs the files created by last week's driver. There are a number of ways to transfer files from one computer to another, but to save you time, the driver can download the files from here.
- Start BlueJ and open the lab2_5a project.
- Click the New Class button and name this class
GreeterPrinter
. Delete all the code and replace it with this
public class GreeterPrinter
{
public static void main(String[] args)
{
}
}
- Now we will add code inside the main method. First let's construct a Greeter object and call its
sayHello
method, and print the result. Here is the code to add to the main method.
Greeter greeter1 = new Greeter();
System.out.println(greeter1.sayHello());
Compile the code and then run by right clicking on the GreeterPrinter class and selecting void main(String[] args)
.Scribe: What is printed on the terminal window?
- Now construct a Greeter object using the constructor that takes a parameter. Like this:
Greeter greeter2 = new Greeter("Mary Programmer");
You can substitute your name.
- Next write the code to call the
sayHello
method of greeter2
and print the result. Compile and run the program.Scribe: What is printed?
- Now on
greeter2
, call the sayGoodbye
method and print the result. Compile and run the program.Driver: What is your code for the main method now?
- Now let's work with Strings. To the end of your main method, add code to create a String like this. (You can use your own name)
String string1 = "Hello, Mary Programmer";
- Add code to call the
length
method of string1 and then print the length.
System.out.println(string1.length());
Scribe: What is printed?
- Add code to call
toUpperCase()
on string1
and print it. Driver: What code did you add?
- Now write the code to print string1.
System.out.println(string1);
or
System.out.println(string1.toString());
Scribe: What is printed? Why isn't it uppercase? This is important. If you do not know, ask your lab instructor.
-
Add the code to call the
replace
method to replace the "e" with "3"
and save the result in a variable called changed
. Remember that the replace method is called something with 2 String parameters.
- Now write the code to print the variable
changed
. Run the program.Driver: What is the code?
- Now write the code to print the variable
string1
Run the programDriver: Paste the output into your report
- Scribe: Explain why
string1
has the value that it does.
Part B
- Now we are going to work with the
Rectangle
class of the Horstmann graphics library in the videos. We will write code to manipulate rectangles. Launch BlueJ and open the lab2_5b project. (The driver can download the files from here, if necessary.)
- Click the New Class button and name the class
RectangleViewer
. Delete all the code and replace it with this
public class RectangleViewer
{
public static void main(String[] args)
{
Rectangle r1 = new Rectangle(5, 10, 20, 30);
r1.draw();
}
}
- Now right-click on the
RectangleViewer
class and select void main(String[] args)
. Click OK. What happens?
- Write the code to construct another rectangle, so that both of them are positioned like this:
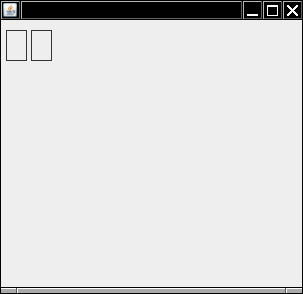
Driver: How did you construct the second rectangle?
- Write the code to call the
translate
method on the first rectangle with parameters 100 and 50. What happens?
- Call the
grow
method on the first rectangle with parameters 50 and 25. What happens?
- How can you get the rectangle back to the original size? (Hint: If you add 50 to a number, you have to subtract 50 to get the original number again.)
- Driver: What is the code in your class?
Part C
It's time for more robots. We will do this in code
- Open the project
lab2_5c
. (The driver can download the files from here, if necessary.)
- Make a new class RobotDemo. Remove the code inside public class RobotDemo and instead put in a main method:
public static void main(String[] args)
. Compile to make sure you did it right.
- Put in the code to construct a
Robot
:
Robot robbie = new Robot();
This constructs a Robot with the image robot.png
On the next line, type robbie
and a period. Then hit Ctrl+Space. Scribe:What happens?
On the Mac, really use the Control key not Command
- This feature is called autocompletion. It is extremely useful because it means you don't have to remember the exact names of all methods. Find a method that makes the Robot move and select it. You still need to add the semi-colon. Scribe: What is the method called?
- Now type
String name = "Robbie Robot";
On the next line, type name
and a period. Then hit Ctrl+Space. What happens?
- With the popup still visible, type
t
(Hit Ctrl-Space again if the popup is gone.). What happens?
- Look at the popup again. Notice exactly how the method to get the uppercase version of a string is spelled. Remember that case is important. Scribe: What is the method name?
- The beauty of autocompletion is that you won't ever have to remember whether it's
toUppercase
or toUpperCase
or touppercase
- On to something more interesting. Remove the
name
statement. and instead figure out how to make the robot turn. How did you do that? (Hint: autocompletion)
- Make the robot move, turn right, move, turn right, and move and turn right another two times (total of 4 right turns). You can just copy/paste the instructions. Run your program. Scribe: What happens?
- Driver: What is the source code of the program? Paste it into your report
- This robot looks a little weird when it turns. It would be better to construct the robot with a different image, such as this one:

To
construct a Robot using an image other than robot.png, you have to use a different constructor. A second constructor lets you set the
(x,y) location of the Robot as well as specify an image.
To construct a robot from this image at location (0, 0), replace the current code that creates the Robot with this
Robot robbie = new Robot(0, 0, "roomba.png");
(The image is in the files you downloaded previously. roomba.png)
- Now change your program again so that the Robot is constructed at location (2, 1) with the roomba.png image and run it. Scribe: What happens? Driver: What is the code for the constructor now?
- It is important to be able to look at the documentation for an unfamiliar class.
I might not be here to tell you how to use the constructor. So do this: Select Tools -> Project Documentation when the workbench has the focus. Scribe: What happens?
If nothing happens, open your browser to the
lab2c/doc/index.html
file, or click
here
Or you can open the class you want and select documentation in the upper right drop-down menu.